建议改名游记.jpg
课程来源。
Filter
filter是个接口。
可以做权限检查、日记操作、事务管理……比如某个文件夹下的东西只有登录了才可以访问之类的。
1 2 3 4 5 6 7 8 9 10 11 12
| public class AdminFilter implements Filter { @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException { HttpSession session = ((HttpServletRequest) servletRequest).getSession(); Object user = session.getAttribute("user"); if (user==null) { servletRequest.getRequestDispatcher("/login.jsp").forward(servletRequest, servletResponse); } else { filterChain.doFilter(servletRequest, servletResponse); } } }
|
然后web.xml里头照猫画虎:
1 2 3 4 5 6 7 8
| <filter> <filter-name>AdminFilter</filter-name> <filter-class>com.atguigu.fliter.AdminFilter</filter-class> </filter> <filter-mapping> <filter-name>AdminFilter</filter-name> <url-pattern>/admin/*</url-pattern> </filter-mapping>
|
除了目录匹配,精准匹配,还有后缀名匹配。用法显然。
生命周期也是启动的时候init,每次doFilter,结束的时候destory。
就好像Servlet有ServletConfig,Filter也有FilterConfig。可以类比第二篇札记内容。
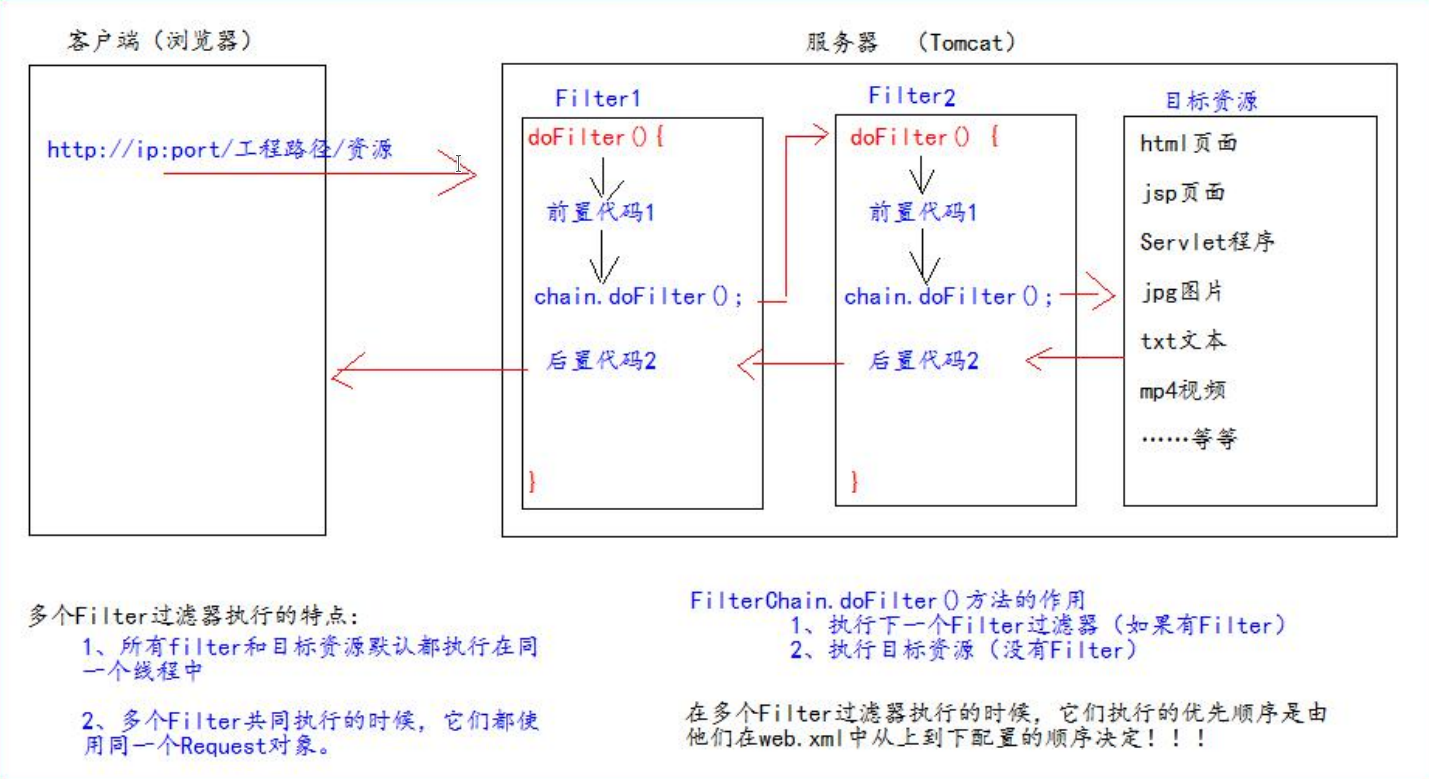
重点是右下角蓝字。
过滤器并不关心请求的资源是否存在。
JSON
json对象和字符串互转:
1 2 3 4 5 6 7 8 9 10 11
| <script type="text/javascript"> var jsonObj = { "key1": 12, "key2": "abc" }; var jsonStr = JSON.stringify(jsonObj); alert(jsonStr); var jsonObj2 = JSON.parse(jsonStr); alert(jsonObj2.key1); alert(jsonObj2.key2); </script>
|
值可以是对象,数组,map……之类的。
和JavaBean互转:
1 2 3 4 5 6 7 8 9 10
| public class JsonTest { public static void main(String[] args) { Person person = new Person(1, "poorpool"); Gson gson = new Gson(); String jsonStr = gson.toJson(person); System.out.println(jsonStr); Person person1 = gson.fromJson(jsonStr, Person.class); System.out.println(person1); } }
|
要用到Gson.jar。
也可以实现list和json互转,map和json互转。不写了。
AJAX
用例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="pragma" content="no-cache" /> <meta http-equiv="cache-control" content="no-cache" /> <meta http-equiv="Expires" content="0" /> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript"> function ajaxRequest() {
var xmlHttpRequest = new XMLHttpRequest();
xmlHttpRequest.open("GET", "http://localhost:8080/13_cookie/ajaxServlet?action=javascriptAjax", true);
xmlHttpRequest.onreadystatechange = function () { if (xmlHttpRequest.readyState == 4 && xmlHttpRequest.status == 200) { var jsonObj = JSON.parse(xmlHttpRequest.responseText); document.getElementById("div01").innerHTML = xmlHttpRequest.responseText; } };
xmlHttpRequest.send(); } </script> </head> <body> <button onclick="ajaxRequest()">ajax request</button> <div id="div01"> d </div> </body> </html>
|
1 2 3 4 5 6 7 8 9
| public class AjaxServlet extends BaseServlet { protected void javascriptAjax(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { System.out.println("收到ajax请求"); Person person = new Person(1, "poorpool"); Gson gson = new Gson(); String jsonStr = gson.toJson(person); resp.getWriter().write(jsonStr); } }
|
使用jquery:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <script type="text/javascript"> function ajaxRequest() { $.ajax({ url: "http://localhost:8080/13_cookie/ajaxServlet", data: {action: "javascriptAjax"}, type: "GET", success: function (msg) { alert("sir yes sir"); $("#div01").html(msg.id + "," + msg.name); }, dataType: "json" }); } </script>
|
非常方便。
有更进一步的封装,$.get(url, data, callback, dataType)
和$.post(...)
,顾名思义。参数变成了url, data, callback和dataType。
更进一步是$.getJSON
,显然是get一个json。参数变成了url, data和callback。
如果你想用ajax做提交表单之类的功能,可以考虑使用$("#form01").serialize()
之类的东西。序列化可以将表单项变成name=value&name=value
。
tomcat 只会将 post 的请求的数据封装到 map 里头,put 都不会。可以自己配了 httpmethodfilter 以后加上 _method
,也可以再配一个 httpputformcontentfilter。